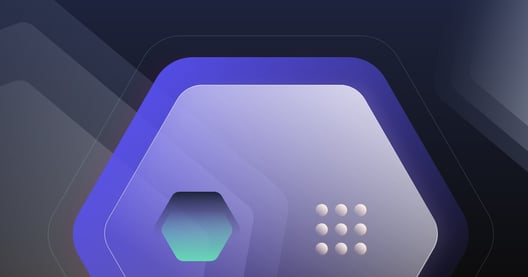
- By Emmanuel Valverde
- ·
- Posted Jan 21, 2023 9:00:00 AM
ATM machine
Iteration 1 Business rules We want to build an ATM machine and the first thing we need to do, is to create the software that will breakdown which..
In computer science, a stack is a famous abstract data type that provides certain operations on a collection of elements. Stacks have a long history, dating back to the very first computer programs and were first documented in 1946.
While you may never need to build a stack yourself, you probably interact with one in your everyday programming in the form of a call stack, so it seems like an important concept to learn about.
Building stacks also provides an opportunity to practise our baby steps in test driven development.
For this kata, build a stack that supports these operations:
Whilst building out these operations, think about the simplest way possible first. Don't go for what you think might be the final implementation right away.
Here are some more important details to consider while building your stack. You could even think of them as iterative requirements along with your operations:
You may recognise one of these requirements as the origin of the famous phrase “Stack Overflow”, which also serves as the name of a certain website you probably look at everyday.
After you have completed building your stack and if you want to go a bit further, try the following.
Your stack currently has protection for a capacity of 0. This is also known as a 'null stack' and a stack with more than 0 is known as a 'bounded stack'. Do you really need all that other code if you receive the request to create a null stack?
There are ways in programming to provide fixed behaviour for known situations. For example, you know for a null stack it will always be empty, always overflow when a push is attempted, and always underflow when a pop is attempted.
Instructions
If the language you are using supports polymorphism, come up with a way to update the creation of a stack, so that it creates a null or bounded stack based on the requested capacity. Then you can add only the code you need to each situation. Your tests should remain the same, but the underlying behaviour has changed.
Help
More languages support polymorphism than you think. Staticly typed languages like C# and Java provide automatic safe polymorphism, by preventing you from messing around with memory and making use of subtyping. In a language like C, that lets you control memory reference, you can do polymorphism using function pointers. You can even do polymorphism in a dynamically typed language like JavaScript and Python, the types are just hidden!
If you are still stuck and you're confused about all this polymorphism, you can find an example of how to solve this requirement in Java here, but only look at this if you have attempted it yourself.
Iteration 1 Business rules We want to build an ATM machine and the first thing we need to do, is to create the software that will breakdown which..
There is a shortage of Christmas trees this year, however, you can help! In the absence of real trees, Santa is going to teach the children of the..
The goal of this Christmas themed kata is to print the lyrics to the 'Twelve Days of Christmas' song, with the smallest amount of lines possible.
Join our newsletter for expert tips and inspirational case studies
Join our newsletter for expert tips and inspirational case studies