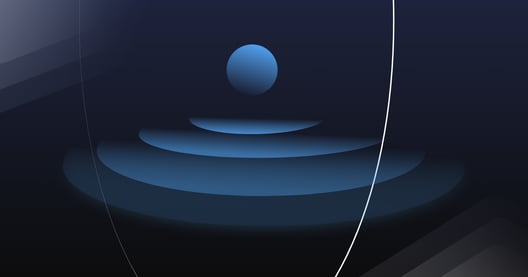
- By Emmanuel Valverde
- ยท
- Posted Mar 5, 2024 12:05:53 PM
Morning Routine
As a forgetful person, I need to remember my morning routine. Because of this, I have created a program that, depending on the time of day, will tell..
We are building a shopping cart for an online grocery shop. The idea of this kata is to build the product in an iterative way.
Name | Cost | % Revenue | Price per unit | Tax | Final price |
Iceberg ๐ฅฌ | 1.55 โฌ | 15 % | 1,79 โฌ | Normal (21%) | 2.17 โฌ |
Tomato ๐ | 0.52 โฌ | 15 % | 0.60 โฌ | Normal (21%) | 0.73 โฌ |
Chicken ๐ | 1.34 โฌ | 12 % | 1.51 โฌ | Normal (21%) | 1.83 โฌ |
Bread ๐ | 0.71 โฌ | 12 % | 0.80 โฌ | First necessity (10%) | 0.88 โฌ |
Corn ๐ฝ | 1.21 โฌ | 12 % | 1.36 โฌ | First necessity (10%) | 1.50 โฌ |
Discounts code | Amount |
---|---|
PROMO_5 | 5% |
PROMO_10 | 10% |
As a customer
I want to see my shopping cart
--------------------------------------------
| Product name | Price with VAT | Quantity |
| ----------- | -------------- | -------- |
|------------------------------------------|
| Promotion: |
--------------------------------------------
| Total products: 0 |
| Total price: 0.00 โฌ |
--------------------------------------------
As a customer
I want to add Iceberg ๐ฅฌ to my shopping cart
I want to add Tomato ๐ to my shopping cart
I want to add Chicken ๐ to my shopping cart
I want to add Bread ๐ to my shopping cart
I want to add Corn ๐ฝ to my shopping cart
I want to see my shopping cart
--------------------------------------------
| Product name | Price with VAT | Quantity |
| ----------- | -------------- | -------- |
| Iceberg ๐ฅฌ | 2.17 โฌ | 1 |
| Tomato ๐
| 0.73 โฌ | 1 |
| Chicken ๐ | 1.83 โฌ | 1 |
| Bread ๐ | 0.88 โฌ | 1 |
| Corn ๐ฝ | 1.50 โฌ | 1 |
|------------------------------------------|
| Promotion: |
--------------------------------------------
| Total products: 5 |
| Total price: 7.11 โฌ |
--------------------------------------------
As a customer
I want to add Iceberg ๐ฅฌ to my shopping cart
I want to add Iceberg ๐ฅฌ to my shopping cart
I want to add Iceberg ๐ฅฌ to my shopping cart
I want to add Tomato ๐ to my shopping cart
I want to add Chicken ๐ to my shopping cart
I want to add Bread ๐ to my shopping cart
I want to add Bread ๐ to my shopping cart
I want to add Corn ๐ฝ to my shopping cart
I want to see my shopping cart
--------------------------------------------
| Product name | Price with VAT | Quantity |
| ----------- | -------------- | -------- |
| Iceberg ๐ฅฌ | 2.17 โฌ | 3 |
| Tomato ๐
| 0.73 โฌ | 1 |
| Chicken ๐ | 1.83 โฌ | 1 |
| Bread ๐ | 0.88 โฌ | 2 |
| Corn ๐ฝ | 1.50 โฌ | 1 |
|------------------------------------------|
| Promotion: |
--------------------------------------------
| Total products: 8 |
| Total price: 12.33 โฌ |
--------------------------------------------
As a customer
I want to add Iceberg ๐ฅฌ to my shopping cart
I want to add Iceberg ๐ฅฌ to my shopping cart
I want to add Iceberg ๐ฅฌ to my shopping cart
I want to add Tomato ๐ to my shopping cart
I want to add Chicken ๐ to my shopping cart
I want to add Bread ๐ to my shopping cart
I want to add Bread ๐ to my shopping cart
I want to add Corn ๐ฝ to my shopping cart
I want to apply my coupon code PROMO_5
I want to see my shopping cart
--------------------------------------------
| Product name | Price with VAT | Quantity |
| ----------- | -------------- | -------- |
| Iceberg ๐ฅฌ | 2.17 โฌ | 3 |
| Tomato ๐
| 0.73 โฌ | 1 |
| Chicken ๐ | 1.83 โฌ | 1 |
| Bread ๐ | 0.88 โฌ | 2 |
| Corn ๐ฝ | 1.50 โฌ | 1 |
|------------------------------------------|
| Promotion: 5% off with code PROMO_5 |
--------------------------------------------
| Total products: 8 |
| Total price: 11.71 โฌ |
--------------------------------------------
You could change this API this is only for example purposes.
Approach 1 passing objects as arguments could be DTO
public interface ShoppingCart {
public void addItem(Product product);
public void deleteItem(Product product);
public void applyDiscount(Discount discount)
public void printShoppingCart();
}
public interface ShoppingCart {
public void addItem(String productName);
public void deleteItem(String productName);
public void applyDiscount(Double discount)
public void printShoppingCart();
}
Approach 3 passing primitives as arguments and returning a DTO
public interface ShoppingCart {
public void addItem(String productName);
public void deleteItem(String productName);
public void applyDiscount(Double discount)
public ShoppingCartList getShoppingCart();
}
The graphic examples that you can see in the assignment are not there to be implemented if you don't want to. They are provided as a reference of how the shopping cart works
As a forgetful person, I need to remember my morning routine. Because of this, I have created a program that, depending on the time of day, will tell..
Inspired by the creative works of Gawain Hewitt: https://gawainhewitt.co.uk/
Iteration 1 Business rules We want to build an ATM machine and the first thing we need to do, is to create the software that will breakdown which..
Join our newsletter for expert tips and inspirational case studies
Join our newsletter for expert tips and inspirational case studies