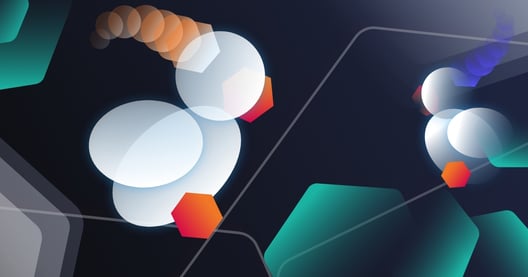
- By Rowan Lea
- ·
- Posted Jan 25, 2024 10:21:11 AM
Songbird
Inspired by the creative works of Gawain Hewitt: https://gawainhewitt.co.uk/
As a forgetful person, I need to remember my morning routine. Because of this, I have created a program that, depending on the time of day, will tell me what I should be doing:From 06:00 to 06:59 - Do exercise
From 07:00 to 07:59 - Read and study
From 08:00 to 08:59 - Have breakfast
This kata could be done with two approaches:
As a forgetful person,
I want a program that reminds me of my morning routine,
So that I can stay on track with my activities.
Given the current time is between 06:00 and 06:59
When I request the routine activity
Then the system should display "Do exercise"
Given the current time is between 07:00 and 07:59
When I request the routine activity
Then the system should display "Read and study"
Given the current time is between 08:00 and 08:59
When I request the routine activity
Then the system should display "Have breakfast"
Given the current time is before 05:59 or after 09:00
When I request the routine activity
Then the system should display "No activity"
Now I'd like to be able to add things to do, that take less than an Hour as well as update the list of things to do for example:From 06:00 to 06:59 - Do exercise
From 07:00 to 07:29 - Read
From 07:30 to 07:59 - Study
From 08:00 to 08:59 - Have breakfast
From 06:00 to 06:44 - Do exercise
From 06:45 to 06:59 - Take a shower
From 07:00 to 07:29 - Read
From 07:30 to 07:59 - Study
From 08:00 to 09:00 - Have breakfast
interface MorningRoutine {
String whatShouldIDoNow();
}
interface MorningRoutine {
void whatShouldIDoNow();
}
interface MorningRoutine {
void whatShouldIDoNow();
}public class MyMorningRouting implements MorningRoutine {
public void whatShouldIDoNow() {
LocalDateTime now = LocalDateTime.now();
int currentHour = now.getHour();
if (currentHour == 6) {
System.out.println("Do exercise");
} else if (currentHour == 7) {
System.out.println("Read and study");
} else if (currentHour == 8) {
System.out.println("Have breakfast");
} else {
System.out.println("No activity");
}
}
}
Inspired by the creative works of Gawain Hewitt: https://gawainhewitt.co.uk/
What do we want to build? We are building a shopping cart for an online grocery shop. The idea of this kata is to build the product in an iterative..
Iteration 1 Business rules We want to build an ATM machine and the first thing we need to do, is to create the software that will breakdown which..
Join our newsletter for expert tips and inspirational case studies
Join our newsletter for expert tips and inspirational case studies