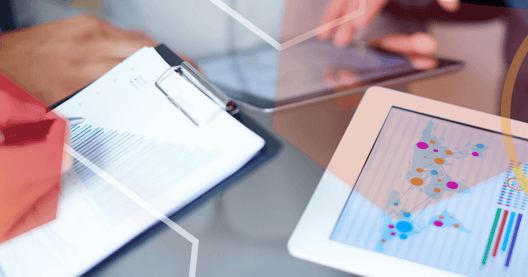
- By Patricia Bourrillon & María Dueñas
- ·
- Posted 20 May 2024
SaaS tools for improving productivity
Can using external tools help improve my team's productivity? This is a very common question among software development team leaders. The answer is a..
Test Driven Development (TDD) has been adopted across developers and teams to deliver quality code through short feedback cycles in a (fail-pass-refactor) fashion. Kent Beck popularized the methodology that became a standard for the industry to follow and build upon. Therefore, starting with TDD is not easy and keeping it sharp so the test suite runs fast is even more complicated. Codebases that tackle business problems require a non-trivial amount of code, and with that, a non-trivial set of test cases. Therefore, as this experience report describes, the industry has an immature test automation process leading to slow feedback.
At Codurance, we are aware of that, and we try to push the industry forward. For example, in Codurance Talks episode 31, Javier Martínez Alcántara, Alasdair Smith and Jose Enrique Rodríguez Huerta discuss building a testing culture.
Along those lines, James Carr came up with a list of anti-patterns to look at and keep under control to avoid the testing trap that extensive codebases might fall into (thus having slow feedback). Dave Farley went through a few of them on his youtube channel, and as such, it made me realize that I had to spread the word on this subject. This post is inspired by Dave Farley's video, a companion for the meetup hosted at Codurance around TDD anti-patterns and a way to share the data from the TDD anti-patterns survey conducted before the meetup.
Along with the meetup, we surveyed to gather data around the TDD-antipatterns in the industry, and from practitioners worldwide. We got 22 answers, and the data shows that practitioners who answered the survey worked for projects in Latin America: Argentina, Brazil, and Mexico and in Europe: France, Hungary, Ireland, Portugal, Spain and Romania.
We also found that the most popular programming languages that respondents work with professionally are:
You will find that most of the examples used in the following sections are in javascript as well. We think this way will be easier to reach a broader audience and give back to those that answered the survey. On the other hand, we also see other languages showing up in the survey that are not as popular: Ruby, Rust and Groovy.
We also found that practitioners usually informally learn TDD, meaning that from the responses, more than 50% of them learned TDD alone through video courses, books or tutorials. Following the same trend, only 50% of the companies understand the pros and cons of TDD and use it as a practice.
According to the survey data, the Excessive setup is the 3rd most popular tdd anti-patterns.
I relate to excessive setup due to the non-practice of TDD from the start and also the lack of practising object callisthenics - it would also apply for functional programming. Still, for the time being, I will stay with object-oriented programming.
The classic approach for excessive setup is when you want to test a specific behaviour on your codebase. It becomes difficult due to the many dependencies you have to create beforehand (such as classes, operating system dependencies, databases - basically anything that removes the attention to the testing goal).
The following code depicts the nuxtjs framework test case for this matter, the test file for the server starts with a few mocks, and then it goes on till the method beforeEach that has more setup work (the mocks).
The code above points to a few dependencies that the test case is mocking to take more fine-tuned control, then the code follows:
At this stage, we know that we are creating a nuxt application and injecting different mocks. So the next bit glues everything together:
Reading the test from the beginning gives an idea that to start with, there are 13 jest.mock invocations. Besides that, there are more setup on the beforeEach, around nine spies and stub setup. Probably, if I wanted to create a new test case from scratch or move tests across different files, I would need to keep the excessive setup as it is now - you know, the excessive setup.
The excessive setup is a common trap. I also felt the pain of having to build many dependencies before starting testing a piece of code. The following code is from my research project called testable - it's a reactjs piece of code that is oriented to functional style:
The excessive number of parameters to test the function are so many that if anyone (or myself) starts to write a new test case, we would forget what to inject into the function to receive the desired result.
Dave Farley shows another example from Jenkins, an open-source ci/cd project. He depicts a particular test case that builds up a web browser to assert the URL being used is a development one:
He argues that the test case creates a web client for asserting the URL, which could have been a regular object call and assert on the returned value, avoiding the web client creation.
The excessive setup can show in different contexts. For a given situation, the question is: are we focused on testing the piece of code that we need or are we spending time setting up the scenario? If the answer is towards the second option, then you have a candidate of being excessive.
The liar is the 4th most popular tdd anti-patterns according to the survey data.
The liar is one of the most common anti-patterns that I can recall in my professional life practising TDD. I would list at least those two reasons for me to spot such issues among codebases:
The first one is well explained in the official jest documentation. Testing asynchronous code becomes tricky as it is based on a future value that you might receive or might not. The following code is a reproduced example from jest documentation depicting the problem related to async testing.
Getting back to the anti-pattern, this test would pass without complaining, you know, the liar. The correct approach is to wait for the async function to finish its execution and give jest control over the flow execution again; this is done by invoking a parameter that jest injects when running the tests. In the code example that follows, this parameter is called done.
The second one, Martin Fowler, elaborates on the reasons for that to be the case, and here I would like to share some opinions that go along with what he wrote.
Asynchronous is a source of non-determinism, and we should be careful with that (as we just explored in the previous example). Besides that, another candidate for such non-determinism are threads.
On the other hand, time-oriented tests sometimes can fail for no reason. For example, I have experienced test suites that failed because the test wasn't using a mock to handle dates. As a result, on the day that the code was written, the test was passed, but on the following day, it broke. You know, the liar again.
According to the survey data, the giant is the 5th most popular tdd anti-patterns.
The giant is also a sign of lack in the design of the codebase. Designing code is also a subject of discussion among TDD practitioners. Sandro Mancuso discussed the relationship between TDD and good design - Does TDD Really Lead to Good Design?
As opposed to the excessive setup, I would say that this anti-pattern can also happen while developing in a TDD fashion. For example, Javier Chacana explored the TPP(Transformation Priority Premise) concepts that show small transformations done in the source code to avoid mirroring the test code with the production code. The giant is often related to the God class, which goes against SOLID principles.
In TDD, the giant often relates to many assertions in a single test case, as Dave Farley depicts in his video. The same test file used from nuxtjs in the excessive setup shows signals of the giant. Inspecting the code closer, we see fifteen assertions for a single test case:
The point of attention here is to reflect if breaking down each block of code and assertion on its test case makes sense. It would require further inspection to double-check if it's possible to break the code as suggested. As Dave Farley depicts in his video, it makes an excellent example of the giant that this practice is not recommended.
According to the survey data, the slow poke is the 6th most popular tdd anti-patterns. Slow poke reminds me of pokemon, and like the creature, the slow poke takes down the efficiency of the test suite. Usually, it puts the test suite to execution and takes longer to finish and give the developer feedback.
One cause for that is time-related code, which is difficult to handle under a test case; It involves manipulating it in different ways.
For example, if we are dealing with payment systems, we would like to trigger some routine to launch a payment at the end of the month. For that, we would need a way to handle time and check for a specific date (the last day of the month) and time (somewhere around the morning? Evening?). In other words, we need a way to handle the time and deal with it without the need of waiting until the end of the month to run the test - or even worse, would you leave your test running for a month to get feedback?.
Code related to time leads to non-determinism, as already mentioned in the "The liar" section. But, lucky us, there is a way to overcome this situation with mocking.
Pushing towards integration tests or end-to-end tests can also transform the test suite into a pain to run, taking long hours or even days. This approach is also related to the ice cream cone instead of the pyramid of the test. In an ideal scenario, you would have as the base more unit tests, a few integration tests and even less from end-to-end tests.
In my experience, the slow poke comes in two different flavours:
Testing and TDD is a practice that developers have adopted and practised for a few years now. Therefore there is still room for improvement. Starting from the anti-patterns that were explored here. In short:
James Carr enumerated 22 anti-patterns, and there is no source of truth, as in comparison, we can find different numbers for different sources. Here, we went over four of them, which means that there are a lot more to go over; each anti-pattern in the list can be explored in different contexts and programming languages - the context is important for those anti-patterns.
Besides that, we also got some information about the practitioners in the field, which anti-patterns they know the most, which programming languages they use. Hopefully, we also leave some insights on how to move forward with this subject.
Keep it sharp, and keep testing!
Can using external tools help improve my team's productivity? This is a very common question among software development team leaders. The answer is a..
You have reached the highest level. You have completed the learning phases and we move on to the more complex katas. Remember that in the link of..
Our recommendation is that you first go through Object-Oriented Programming katas so that you can move on to more complex testing concepts. If so, we..
Join our newsletter for expert tips and inspirational case studies
Join our newsletter for expert tips and inspirational case studies